Summary: in this tutorial, you will learn how to create the React Hello World web application.
Prerequisites
Before you start working with ReactJS, you need to have Node.js and Node Package Manager (npm) installed on your computer.
First, open the command prompt on Windows or Terminal on Unix-like systems.
Second, run the following command to check the version of Node.js:
node --version
Code language: plaintext (plaintext)
Output:
v21.3.0
Code language: plaintext (plaintext)
Creating a React project
First, open a terminal and run the following command:
npx create-react-app hello-world
Code language: plaintext (plaintext)
This command will create a new directory named hello-world
and set up the React project files and directories. It’ll take a few minutes because the command will create many files and directories.
Second, navigate to the project directory:
cd hello-world
Code language: plaintext (plaintext)
Third, run the following command to start the React development server:
npm start
Code language: plaintext (plaintext)
This command will start the development and run the React application. It’ll launch the URL http://localhost:3000 in the web browser:
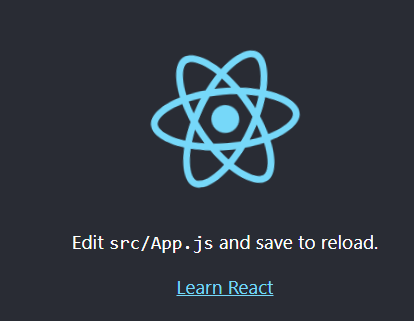
Finally, stop the development server by pressing the Ctrl-C
on the terminal:
...
^CTerminate batch job (Y/N)? Y
Code language: plaintext (plaintext)
It’ll prompt you to enter the letter Y (yes) or N (no) to confirm whether you want to stop the development server. Input Y and press the Enter key to confirm.
Examining files and directories of the React project
Open the project directory in your favorite editor. Here’s the React project directory in VS Code:
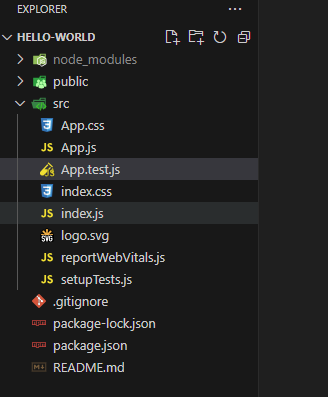
Typically, you’ll often work with the files in the src
directory. Besides CSS and SVG files, the src
directory includes the following JavaScript files:
- App.js
- index.js
- reportWebVital.js
- setupTests.js
These JavaScript files contain the code (JavaScript & JSX code) that cannot be executed directly in the web browser. We’ll discuss JSX shortly.
Instead, the React development server needs to transpile the code in these files to the native JavaScript code that the web browser can execute.
To do that, React Development Server uses the following tools:
- Babel to transpile the JavaScript code.
- Webpack tool to merge the output JavaScript files into a single file called bundle.js
The following picture illustrates the process:
If you view the source code of the react app at localhost:3000, you’ll see the bundle.js file:
<script defer src="/static/js/bundle.js"></script>
Code language: HTML, XML (xml)
The following picture illustrates how the process works:
First, the web browser requests the index.html
file from localhost:3000
React Development Server. The index.html
includes the index.js
file.
Second, the React Development Server uses Babel to transpile the code in the .js files into native JavaScript code and Webpack to merge these files into the bundle.js
file.
Third, the React Development server responds to the request by delivering the bundle.js
file to the web browser.
The project directory has the following files and directory required for the React application to run properly:
Files/Directories | Description |
---|---|
src/index.js | This is the first file that executes when the React app starts. |
public/index.html | This is the skeleton for the React app. |
package.json | Provide a list of dependencies the React app needs. The project also includes the package.json.lock file. |
node-modules | Store the dependencies of the React app. |
To create a page that displays the Hello, World! message in React, we’ll delete all files in the public
directory except the index.html
file and all the files in the src
directory. Additionally, we’ll create a completely new index.js file.
Creating React Hello World app
Here’s the complete code of the index.js
file:
import React from 'react';
import ReactDOM from 'react-dom/client';
const el = document.querySelector('#root');
const root = ReactDOM.createRoot(el);
function App() {
return <h1>Hello, World!</h1>;
}
root.render(<App />);
Code language: JavaScript (javascript)
How it works.
First, import React libraries:
import React from 'react';
import ReactDOM from 'react-dom/client';
Code language: JavaScript (javascript)
These react libraries serve different purposes:
- The
React
library allows you to define components and how multiple components work together. - The
ReactDOM
library knows how to display a component in the web browser.
Next, select the root element in the public/index.html
file:
const el = document.querySelector('#root');
Code language: JavaScript (javascript)
If you open the index.html
file, you’ll see the div
element with the root
id:
<div id="root"></div>
Code language: HTML, XML (xml)
Then, instruct React to take control of the root
element by calling the createRoot()
function:
const root = ReactDOM.createRoot(el);
Code language: JavaScript (javascript)
After that, define a React component called App
:
function App() {
return <h1>Hello, World!</h1>;
}
Code language: JavaScript (javascript)
In React, a component is a JavaScript function that returns JSX.
JSX stands for JavaScript XML, an extension of JavaScript that allows you to write HTML-like code directly within JavaScript code.
In this example, the App
component returns the following JSX code:
<h1>Hello, World!</h1>
Code language: HTML, XML (xml)
When you run the Development Server, Babel will transpile this JSX code into the following JavaScript code:
import { jsx as _jsx } from "react/jsx-runtime";
/*#__PURE__*/_jsx("h1", {
children: "Hello, World!"
});
Code language: JavaScript (javascript)
In the output code:
- First, import the jsx as _jsx function from the library react/jsx-runtime.
- Second, call the _jsx function to generate the h1 tag with content.
Note that the output JavaScript code of JSX from Babel may be changed in the future. But its goal remains the same as generating the h1 HTML tag with the text “Hello, World!”.
If you want to view the transpiled version of JSX, you can navigate to the babeljs site, enter the JSX code, and view the output JavaScript code.
Finally, show the React component on the screen:
root.render(<App />);
Code language: HTML, XML (xml)
If you run the development server, you’ll see the message on the screen:
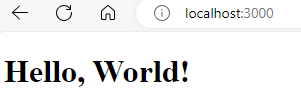
Summary
- A React component is a function that returns some JSX.
- JSX is an extension to JavaScript that allows you to write HTML-like directly inside JavaScript code.
- React Development server uses Babel to transpile JSX code to JavaScript code, and Webpack to merge output files into the bundle.js file for running the React app in the web browser.