Summary: in this tutorial, you will learn how to render JSX elements conditionally using the if statement, logical AND operator (&&), and logical OR operator (||).
Sometimes you may want to display different JSX elements based on a condition. To do that, you can use one of three following techniques:
- if statement or ternary operator (?:).
- Logical AND operator (&&).
- Logical OR operator (||).
We’ll create a simple React application to demonstrate each technique.
Creating a React app
First, open a terminal and run the npx create-react-app
command to create a new react app:
npx create-react-app todo
Code language: plaintext (plaintext)
Second, delete all files in the src
directory.
Third, create an index.js
file in the src
directory. The index.js
file will render the App
component on the screen:
import ReactDOM from 'react-dom/client';
import App from './App.js';
const el = document.querySelector('#root');
const root = ReactDOM.createRoot(el);
root.render(<App />);
Code language: JavaScript (javascript)
Fourth, create an App.js
in the src directory that hosts the App component:
import TodoList from './TodoList';
const App = () => {
const todos = [
{ id: 1, title: 'Learn React', completed: true },
{ id: 2, title: 'Build an app', completed: false },
{ id: 3, title: 'Deploy the app', completed: false },
];
return (
<main>
<h1>Todo List</h1>
<TodoList todos={todos} />
</main>
);
};
export default App;
Code language: JavaScript (javascript)
The App
component passes the array of todo objects to the TodoList
component.
Finally, create the TodoList.js
file to store the TodoList
component:
const TodoList = ({ todos }) => {
const renderedTodos = todos.map((todo) => {
return <div key={todo.id}>{todo.title}</div>;
});
return <section>{renderedTodos}</section>;
};
export default TodoList;
Code language: JavaScript (javascript)
The TodoList
component renders the data from the todo array into div elements.
Here’s the output:
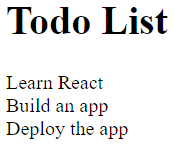
To put a check on a completed todo, you can render each completed todo using one of the above-mentioned techniques.
Conditional rendering using an if statement
The following uses an if
statement to return different JSX elements based on the completed
property of each todo object:
const TodoList = ({ todos }) => {
const renderedTodos = todos.map(({ id, title, completed }) => {
if (completed) {
return <div key={id}>✔ {title}</div>;
}
return <div key={id}>{title}</div>;
});
return <section>{renderedTodos}</section>;
};
export default TodoList;
Code language: JavaScript (javascript)
Output:
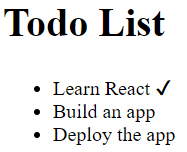
Alternatively, you can use a ternary operator (?:
) to make the code more concise:
const TodoList = ({ todos }) => {
const renderedTodos = todos.map(({id, title, completed}) => {
return (
<div key={id}>
{completed ? '✔' : ''}
{title}
</div>
);
});
return <section>{renderedTodos}</section>;
};
export default TodoList;
Code language: JavaScript (javascript)
In this example, we use the following expression directly within the JSX:
{todo.completed ? '✔' : ''}
Code language: JavaScript (javascript)
If the completed
is true
, then the expression returns the check or an empty string otherwise.
Conditional Rendering using a logical AND operator
The logical AND operator allows you to return the first falsy value or the last truthy value:
const result = value1 && value2;
Code language: JavaScript (javascript)
You can utilize this feature to render elements conditionally in JSX:
const TodoList = ({ todos }) => {
const renderedTodos = todos.map((todo) => {
return (
<div key={todo.id}>
{todo.completed && '✔'}
{todo.title}
</div>
);
});
return <section>{renderedTodos}</section>;
};
export default TodoList;
Code language: JavaScript (javascript)
In this example, if the completed
is false
, the expression returns the first falsy value, which is false. Consequently, React will show an empty string because JSX does not render boolean values (true
and false
)
If the completed
is true
, the expression returns the ‘✔’ because it is the last truthy value. Therefore, React will render the ‘✔’ for completed todos.
Conditional Rendering using a logical || operator
The logical OR operator (||) returns the first truthy value or the last falsy value:
const result = value1 || value2;
Code language: JavaScript (javascript)
Suppose, you want to display the character ‘✖’ in front of incompleted todos. To achieve that, you can use the logical OR operator (||):
{todo.completed || '✖'}
Code language: JavaScript (javascript)
The expression returns true if the completed is true or the character ‘✖’ if the completed is false.
const TodoList = ({ todos }) => {
const renderedTodos = todos.map(({ id, title, completed }) => {
return (
<div key={id}>
{completed && '✔'}
{completed || '✖'}
{title}
</div>
);
});
return <section>{renderedTodos}</section>;
};
export default TodoList;
Code language: JavaScript (javascript)
Output:
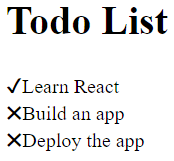
Summary
- Use the if statement to return different JSX elements based on a condition.
- Use the ternary operator, logical AND operator, and logical OR operator to render elements conditionally within JSX