React is a popular, declarative, component-based, state-driven JavaScript library for building user interfaces:
React is popular. React was introduced in 2013 by Facebook and has become one of the most important libraries for building user interfaces (UI).
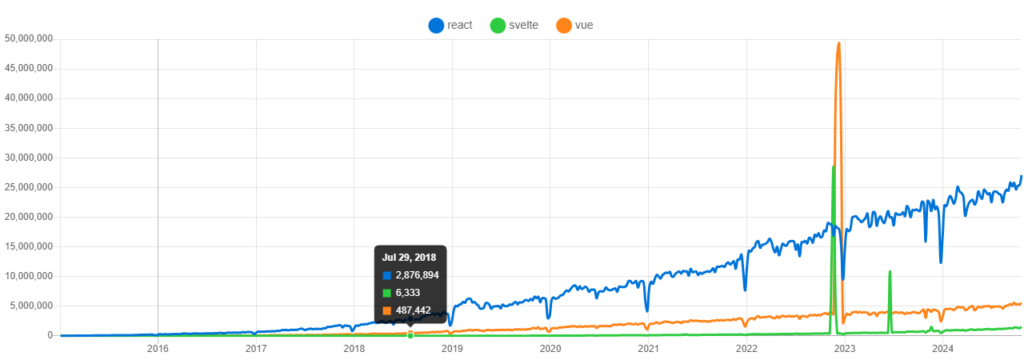
React is declarative. You describe what UI looks like, and React renders and updates it when the data changes:
export const App = () => {
return (
<main>
<NavBar />
<Search />
<List />
</main>
);
};
Code language: JavaScript (javascript)
React is component-based. In a React app, you build UI using small, reusable pieces like Navbar, Search, and List. These are called components in React.
Reat is state-driven. Data that changes over time is called state. React manages the state of your application and updates the UI when the states change:
To learn React quickly, it’s recommended that you write many React applications, starting from simple ones and gradually moving to real-world projects.
Section 1. Getting started with React
This section will help you start with React quickly by explaining how React works.
- React Hello World – Let’s create a simple React app.
- JSX – Learn about JSX and how to write JSX elements properly in a React application
- Props – Learn to pass data from the parent component to child components using the React Props system.
- Key Prop – Show you how to render a list in the React app properly using the
key
prop. - Conditional Rendering – Learn how to render JSX elements based on a condition.
- Events – Guide you on handling events such as mouse clicks or form submissions using the React events.
- State – Show you how to work with React state and events to create interactive React components.
Section 2. Interacting with API
This section shows you how to create a react app that uses an external API.
- React API Call – Show you how to create a Wikipedia search app in React.
Section 3. Todo Apps
In this section, we’ll progressively create three versions of the Todo app in React.
- React Todo App – Learn step-by-step how to create a React Todo App.
- React Todo with API – Create a Todo app that uses API for data persistence.
- React Todo App with Context – Use context to share state across your entire React App.
Section 4. React Hooks in Depth
- useState – Learn to add a state variable to a component using the
useState
hook. - useEffect – Guide you on how to run a side effect function in the component.
- useReducer – Show you how to use the
useReducer
hook to manage multiple closely related states more efficiently. - useRef – Learn how to use the
useRef
hook to access DOM elements directly and modify the state without causing a rerender.
Section 5. Tooling
- React + Tailwind CSS – Show you how to install Tailwind CSS in the React app.