Summary: in this tutorial, you will learn how to place components in the flexbox layout using relative and absolute positions.
Relative positioning
React native uses the flexbox layout to arrange the components on the screen. By default, the position
property of each component is relative
:
{
position: 'relative'
}
Code language: JavaScript (javascript)
The { position: 'relative ' }
means the component is positioned relatively to its original position within the flexbox layout.
React Native allows you to use the top
, right
, bottom
and left
properties to position a component relative to its original position.
For example, the following shows a big green box and a small yellow box:
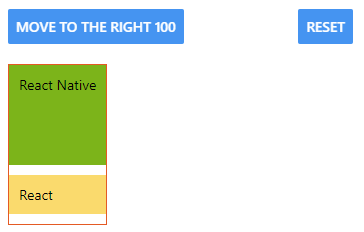
If you click the “Move to the right 100” button, it will move the small yellow box to the right 100 unit from its original position within the flexbox layout:
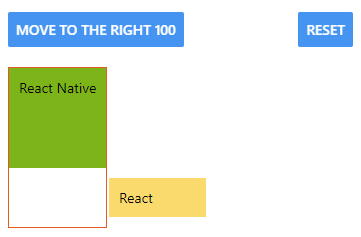
import { View, Text, StyleSheet, SafeAreaView, Button } from 'react-native';
import { useState } from 'react';
const PositionRelative = () => {
const [position, setPosition] = useState({});
return (
<SafeAreaView style={styles.container}>
<View style={styles.app}>
<View style={styles.actions}>
<Button title="Move to the right 100" onPress={() => { setPosition({ left: 100 }); }} />
<Button title="Reset" onPress={() => { setPosition({}); }} />
</View>
<View style={styles.layout}>
<Text style={styles.bigBox}>React Native</Text>
<Text style={[styles.box, position]}>React</Text>
</View>
</View>
</SafeAreaView>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
app: {
flex: 1,
padding: 20,
alignItems: 'flex-start',
},
layout: {
borderColor: '#EE5013',
borderWidth: 1,
},
bigBox: {
marginBottom: 10,
position: 'relative',
padding: 10,
backgroundColor: '#78B500',
height: 100,
},
box: {
marginBottom: 10,
position: 'relative',
padding: 10,
backgroundColor: '#FFD966'
},
actions: {
alignSelf: 'stretch',
flexDirection: 'row',
justifyContent: 'space-between',
marginBottom: 20,
}
});
export default PositionRelative;
Code language: JavaScript (javascript)
Absolute positioning
Besides the relative
position, you can set the position
of a component to absolute
:
{
position: 'absolute'
}
Code language: JavaScript (javascript)
In this case, React Native removes the component from the flexbox flow layout and places it to its closest containing component.
In the following picture, we also have a View
that contains two boxes: a big green box and a small yellow box:
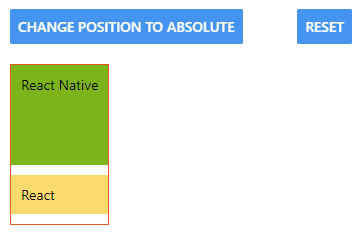
If you click the “Change Position to Absolute” button, it will change the display
property of the small yellow box to absolute
and reposition the component relative to the View
:
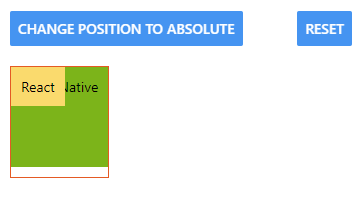
Notice that the position absolute only changes the position of the small yellow box. It doesn’t change other properties of the flexbox like alignItems: 'flex-start'
.
Once you set the position to absolute, you can also control the component’s position using the top
, right
, left
, bottom
properties within the containing component. These properties accept both positive and negative values.
For example, the following shows how to use absolute positioning to create a cart icon with the number of items on it:
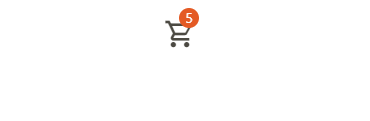
import { StyleSheet, Text, View, SafeAreaView } from 'react-native'
import Icon from '@expo/vector-icons/MaterialCommunityIcons';
const Cart = () => {
return (
<SafeAreaView style={styles.container}>
<View style={styles.app}>
<View style={styles.cart}>
<Icon name="cart-outline" size={32} color="#4B4942" />
<Text style={styles.cartText}>5</Text>
</View>
</View>
</SafeAreaView>
)
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
app: {
flex: 1,
padding: 20,
},
cartText: {
backgroundColor: '#EE5013',
width: 20,
height: 20,
borderRadius: 10,
textAlign: 'center',
color: '#fff',
fontSize: 14,
position: 'absolute',
left: 15,
top: -10
}
});
export default Cart;
Code language: JavaScript (javascript)
Summary
- Use
position
relative
to move the component relative to its original position. - Use
position
absolute
to remove the component from its flexbox layout flow and reposition it to its closest containing component. - Use the
top
,right
,bottom
, andleft
properties to position the component.