Summary: in this tutorial, you will learn about what Node.js is and why and when you should use Node.js.
Introduction to Node.js
Node.js is an open-source cross-platform runtime environment that allows you to use JavaScript to develop server-side applications.
Every web browser has a JavaScript engine that compiles JavaScript code to machine code. For example, Firefox uses SpiderMonkey, and Google Chrome uses V8.
Because browsers use different JavaScript engines, sometimes, you will see that JavaScript behaves differently between the browsers.
In 2009, Ryan Dahl, the creator of Node.js, took the V8 engine and embedded it in an application that could execute JavaScript on the server.
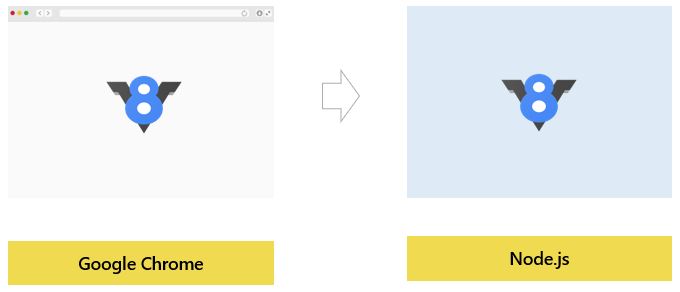
The following picture illustrates the Node.js system:
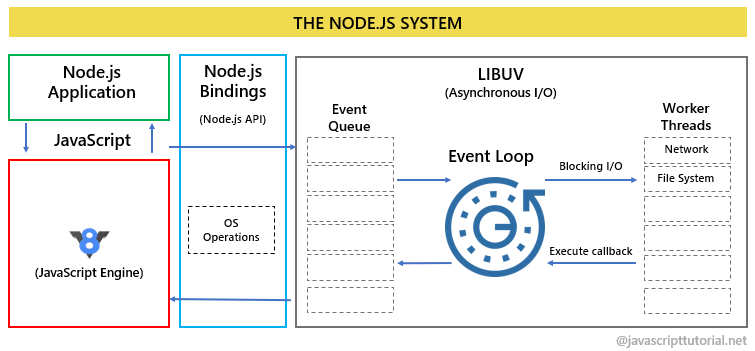
Node.js uses the single-threaded, non-blocking, and event-driven execution model, which is similar to the execution model of JavaScript in the web browser.
Node.js is single-threaded
Node.js is single-threaded, meaning each process runs only one thread of execution.
The single-threaded execution model allows Node.js to handle more concurrent requests easily via the event loop. As a result, Node.js applications typically consume less memory compared to others.
If you are unfamiliar with the event loop, check out this tutorial. It’s important to note that the event loop in Node.js works similarly to the event loop in web browsers.
Node.js uses Non-blocking I/O
I/O stands for input/output, which can involve disk access, a network request, or a database connection. I/O requests are expensive and slow, leading to the potential blocking of other operations
Node.js addresses blocking I/O issues by utilizing non-blocking I/O operations.
In the non-blocking model, you can initiate a request while doing other tasks. Once the request is completed, a callback function is executed to handle the result.
Node.js is event-driven
Node.js uses event-driven architecture, meaning that Node.js waits for events to happen and responds to them when they occur, instead of following a linear, top-to-bottom execution model.
The following describes how Node.js event-driven architecture works:
- An event, such as a client sending a request to a server, triggers an event handler function.
- Node.js then adds the event handler (a callback function) to an Event Queue.
- The Event Loop, which is a continuous loop running in Node.js, checks the Event Queue and processes the events one by one. It executes the corresponding event handler for each event.
- While the Event Loop is processing these events, Node.js can continue handling other tasks, such as listening for new events.
Why node.js
Node.js is good for prototyping and agile development, allowing you to build fast and highly scalable applications.
Node.js has a large ecosystem of open-source libraries so that you can reuse existing ones and spend more time focusing on the business logic.
If you already use JavaScript for front-end development, you can leverage your existing skills for server-side development.
Using the same JavaScript for both client-side and server-side development makes your codebase cleaner and more consistent.
Node.js real-world use cases
Node.js has a wide range of real-world applications:
- Scalable network applications: Node.js is used by big companies like LinkedIn, Uber, and Netflix to develop scalable applications.
- Real-time applications – Since Node.js uses an event-driven, non-blocking I/O model, it is ideal for real-time applications including chat and online gaming.
- API and Microservices – Node.js is commonly used to develop Restful APIs and microservices.
- IoT devices: Node.js powers various Internet of Things (IoT) applications.
Summary
- Node.js is an open-source cross-platform runtime environment that allows you to use JavaScript to develop server-side applications.