Summary: This tutorial helps you get started with JavaScript by showing you how to embed JavaScript code into an HTML page to display the Hello World message.
Creating a JavaScript Hello World project
Step 1. Create a new project directory called helloworld
to store the HTML and JavaScript files.
Step 2. Open the helloworld
project directory in your favorite code editor. We’ll use the VS Code.
Step 3. Create a new HTML file namedindex.html
inside the helloworld
project directory with the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>JavaScript Hello, World!</title>
</head>
<body>
<script>
alert('Hello, World!');
</script>
</body>
</html>
Code language: HTML, XML (xml)
Step 4. Right-click the editor and select “Open with Live Server”. VS Code will start a web server and open the index.html in the default web browser:
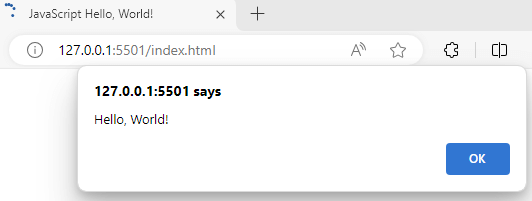
How it works.
First, use the <script>
element to insert JavaScript into the index.html
file:
<script>
alert('Hello, World!');
</script>
Code language: HTML, XML (xml)
Second, call the alert() function to display an alert on the web browser:
alert('Hello, World!');
Code language: JavaScript (javascript)
The alert()
is a built-in function available on a web browser for displaying an alert.
It’s important to note that placing JavaScript code inside the <script>
element directly in HTML is not recommended and should be used only for proof of concept or testing purposes.
A common way to embed JavaScript code in an HTML document is to create a new JavaScript file and use a <script>
tag to reference it.
Including an external JavaScript file
Step 1. Create a new directory in the project directory called js
. By convention, we place the JavaScript files in a directory called js
, which stands for JavaScript.
Step 2. Create a new app.js
file in the js
directory with the following code:
alert('Hello, World!');
Code language: JavaScript (javascript)
Step 3. Modify the index.html
to include the app.js
file before the body
closing tag:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>JavaScript Hello, World!</title>
</head>
<body>
<script src="js/app.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
How it works.
The following includes the app.js
file from the js
directory in the index.html
file:
<script src="js/app.js"></script>
Code language: HTML, XML (xml)
In this syntax, we place the path to the JavaScript file in the src
attribute of the <script>
tag.
When the web browser encounters the <script>
tag, it downloads the app.js
file specified in the src
attribute and executes the JavaScript file.
Summary
- Use
<script>
element to include a JavaScript file in an HTML page. - Use
alert()
function to display an alert in the web browser.