Summary: in this tutorial, you will learn how to display a confirmation dialog by using the JavaScript confirm()
method.
Introduction to JavaScript confirm() method
To invoke a dialog with a question and two buttons OK
and Cancel
, you use the confirm()
method of the window
object:
let result = window.confirm(question);
Code language: JavaScript (javascript)
In this syntax:
- The
question
is an optional string to display in the dialog. - The
result
is a Boolean value indicating whether theOK
orCancel
button was clicked. If theOK
button is clicked, the result istrue
; otherwise, the result isfalse
.
Note that if a browser ignores in-page dialogs, then the result
is always false
.
The confirmation dialog is modal and synchronous. It means that the code execution stops when a dialog is displayed and resumes after it has been dismissed.
The following example uses the confirm()
method to invoke a confirmation dialog. Based on the user’s selection, it displays the corresponding message based using the alert()
method:
let result = confirm('Are you sure you want to delete?');
let message = result ? 'You clicked the OK button' :
'You clicked the Cancel button';
alert(message);
Code language: JavaScript (javascript)
Click here to show the confirmation dialog
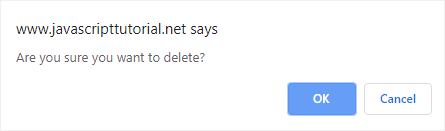
Summary
- The
confirm()
is a method of thewindow
object. - The
confirm()
shows a system dialog that consists of a question and two buttons:OK
andCancel
. - The
confirm()
returnstrue
if theOK
button was clicked orfalse
if theCancel
button was selected.