Summary: in this tutorial, you’ll learn about the Express web framework and how to build an Express Hello World app.
Introduction to the Express
Express is a fast, unopinionated, and minimal web framework for Node.js. Express provides a set of features suitable for building web applications.
With Express, you can:
- Build web applications including single-page and multi-page applications.
- Build an API server.
Benefits of Express
- Simplicity: Express helps you simplify building web servers and APIs by providing a minimalistic approach.
- Flexibility: Express is unopinionated, meaning you can structure your application as you see fit.
- Active community and ecosystem: Express has extensive documentation and third-party libraries that help you develop web apps easier and faster.
Creating the Express Hello World app
Step 1. Open your terminal or command prompt and create a new project directory named express-server
.
mkdir express-server
cd express-server
Code language: plaintext (plaintext)
Step 2. Run the npm init --yes
command inside your project directory to initialize a new Node.js project:
npm init --yes
Code language: plaintext (plaintext)
This command will create a package.json
file where you can manage dependencies and scripts.
Step 3. Install the express
package by running the following npm install
command:
npm install express
Step 4. Set the value for the type
key to "module"
in the package.json
file to instruct Node.js to use ES modules:
"type": "module",
Code language: JSON / JSON with Comments (json)
Additionally, change the scripts
section in the package.json
file to the following:
"scripts": {
"start": "node --watch index.js"
},
Code language: JSON / JSON with Comments (json)
When you run the command npm start
, it’ll execute the node --watch index.js
command. The --watch
flag will restart the server whenever you change the source code.
If you don’t add the --watch
flag, you must restart the server whenever you change the code.
Please note that the --watch
flag has been available in Node.js 19 or later.
Step 5. Create an index.js
file with the following code:
import express from 'express';
const app = express();
const PORT = 3000;
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Code language: JavaScript (javascript)
Step 6. Open the terminal and run the server:
npm start
Code language: plaintext (plaintext)
It’ll show the following message on the terminal:
Server is listening on port 3000
It means that the server is running and listening on the port 3000. If you launch the page http://localhost:3000/
on the web browser, you’ll see the Hello, World!
message on the screen.
How the Express app works
First, import the express
function from the express
library:
import express from 'express';
Code language: JavaScript (javascript)
The express
is a function that returns an instance of an Express
app.
Next, call the express()
function to get an instance of the Express
app:
const app = express();
Code language: JavaScript (javascript)
Then, define a PORT
constant that stores the port number for the server to listen on:
const PORT = 3000;
Code language: JavaScript (javascript)
After that, define a route handler that handles the HTTP GET request to the site route /
app.get('/', (req, res) => {
res.send('Hi there');
});
Code language: JavaScript (javascript)
The get()
method has two parameters:
- The first parameter is the route
/
. - The second parameter is a callback function with two parameters:
req
andres
. Thereq
andres
stand forrequest
andresponse
respectively. This callback function is called a router handler.
The req
represents the HTTP request and the res
describes the HTTP response. They are the instances of the Request
and Response
classes respectively. These objects have many helpful properties and methods for handling HTTP requests and responses.
In this example, we call the send()
method of the HTTP response object to return a simple text.
Finally, instruct the app to listen to requests on the port 3000
:
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Code language: JavaScript (javascript)
Returning JSON to Client from the Express app
The following shows how to return JSON to the client:
import express from 'express';
const app = express();
const PORT = 3000;
app.get('/', (req, res) => {
res.send({ message: 'Hello, World!' });
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Code language: JavaScript (javascript)
In this code, we call the send()
function to return JSON data to the client:
res.send({ message: 'Hello, World!' });
Code language: JavaScript (javascript)
If you open the URL http://localhost:3000/
on the web browser, you’ll see the JSON instead of a simple text.
{
"message": "Hi there"
}
Code language: JSON / JSON with Comments (json)
Adding Environment Variables
Node.js v20.6.0 or later supports .env
files for configuring environment variables via the process
core module.
The process
core module has the env
property that stores all the environment variables. Node.js automatically imports the process
module so you don’t need to import it before use.
Step 1. Create a new .env
file in the project directory and set the PORT
variable to 3000
:
PORT=3000
Code language: plaintext (plaintext)
In the app, you can access the PORT
variable using the proccess
module like this:
process.env.PORT
Code language: plaintext (plaintext)
Step 2. Modify the index.js
file to use the PORT
variable:
import express from 'express';
const app = express();
const PORT = process.env.PORT || 3000;
app.get('/', (req, res) => {
res.status(200).send({ message: 'Hello, World!' });
});
app.listen(PORT, () => {
console.log(`Server is listening on port ${PORT}`);
});
Code language: JavaScript (javascript)
How it works.
First, define the PORT
variable and assign process.env.PORT
to it. If the PORT
environment variable is not available, the PORT
variable will default to 3000
:
const PORT = process.env.PORT || 3000;
Code language: JavaScript (javascript)
Second, use the PORT
in the listen()
method:
app.listen(PORT, () => {
console.log(`Server is listening on port ${PORT}`);
});
Code language: JavaScript (javascript)
You’ll see the same message in the terminal:
Server is listening on port 3000
Code language: plaintext (plaintext)
Using REST Client on VS code
If you use VS Code, you can use the REST Client extension to make HTTP requests and view the response directly within the editor.
Step 1. Install REST Client extension.
Step 2. Create a new file called api.http
in the project directory.
Step 3. Add the following code to make an HTTP GET request to http://localhost:3000/
GET http://localhost:3000/
Content-Type: application/json
Code language: HTTP (http)
Step 4. Click the Send Request to make the HTTP request.
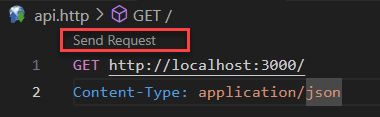
Step 5. View the HTTP response:
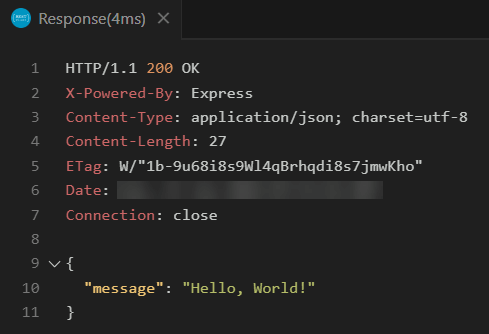
Besides the REST Client tool, you can use other REST client tools like Postman to make HTTP requests.
Downloading the Express Hello World app
Step 1. Download the Express Hello World App:
Download the project source code
Step 2. Extract it to a directory e.g., express-server
Step 3. Open your terminal or command prompt and run the npm install command to install dependencies:
npm install
Step 4. Run the npm start to run the Express app:
npm start
Summary
- Express is a flexible web application framework for building web applications and API.
- Use the REST Client extension to make HTTP requests and view HTTP responses directly within VS Code.
- Use the
process.env
to access the environment variables.